适配器模式(Adapter Pattern) : 该模式是两个不兼容接口的桥梁, 让接口不兼容的类之间也能协同工作, 适配器模式既可以作为类结构型模式, 也可以作为对象结构型模式.
设计模式-概述
结构
适配器模式有如下构成:
- Adapter 目标抽象接口
- ConcreteAdapter 适配器类
- Adaptee 被适配者
- Client 客户类
对象适配器

类适配器
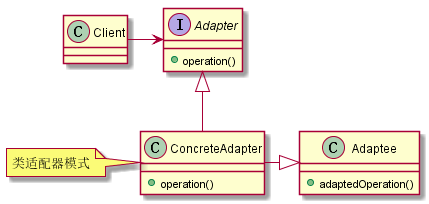
使用时序

- 客户端想要使用Adaptee, 但是它用不了这个接口, 怎么办呢?
- 客户端使用了一个适配器Adapter, 把想要的使用的接口通过参数传入
- 客户端使用Adapter的request方法, Adapter适配了Adaptee的specificRequest()方法.
- 客户端最终获取到结果.
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95
| package com.yuda.test.adapter;
import java.io.File;
public class AdapterDemo { public static void main(String[] args) { Adapter adapter1 = new TencentCosClientAdapter(new TencentCosClient()); Adapter adapter2 = new AliyunOssClientAdapter(new AliyunOssClient());
adapter1.upload(new File("C://test.mp4"));
} }
interface Adapter { void upload(File file); }
interface IAliyunOssClient { void uploadToOss(File file);
void otherOssMethod(Object o); }
interface ITencentCosClient { void uploadToCos(File file);
void otherOssMethod(Object o); }
class AliyunOssClient implements IAliyunOssClient {
@Override public void uploadToOss(File file) { System.out.println("上传到阿里云oss"); }
@Override public void otherOssMethod(Object o) { System.out.println("阿里云Client的其他方法"); } }
class TencentCosClient implements ITencentCosClient {
@Override public void uploadToCos(File file) { System.out.println("上传到腾讯云cos"); }
@Override public void otherOssMethod(Object o) { System.out.println("腾讯云Client的其他方法"); } }
class AliyunOssClientAdapter implements Adapter {
private IAliyunOssClient client;
public AliyunOssClientAdapter(IAliyunOssClient client) { this.client = client; }
@Override public void upload(File file) { client.uploadToOss(file); } }
class TencentCosClientAdapter implements Adapter {
private ITencentCosClient cosClient;
public TencentCosClientAdapter(ITencentCosClient cosClient) { this.cosClient = cosClient; }
@Override public void upload(File file) { cosClient.uploadToCos(file); } }
|
- 版本1, 需要上传文件到腾讯云, 使用了腾讯云sdk, sdk里面有第三方定义的接口
ITencentCosClient
;
- 版本2, 需要上传文件到阿里云, 使用了阿里云sdk, sdk里面有第三方定义的接口
IAliyunOssClient
;
- 后续的版本, 有可能上传到其他云, 使用了其他的sdk, 它的接口又不一样了.
- 为了应对这种变化, 使用适配器模式, 编写适配器, 把它变成自己的接口
Adapter
, 管他是阿猫云, 阿狗云, 阿鸟云…的统统变成我的通用接口.
使用场景
- 系统必须要使用某个类, 但是这个类的接口不是系统想要的, 这个类还不能乱改动.
- 有大量功能类似的类, 他们都有不同的接口, 接口作用类似, 但之间没有任何联系, 系统可能会用到这些类, 甚至其他类似的类.
应用
- Android 里面的列表控件, 需要用到 Adapter 来应对不同的内容, 参考:github-canyuda/SmartBulter-ChatListAdapter.java
- Java语言的数据库连接工具JDBC, JDBC给出一个客户端通用的抽象接口, 每一个具体数据库引擎的JDBC驱动软件都是一个介于JDBC接口和数据库引擎接口之间的适配器.